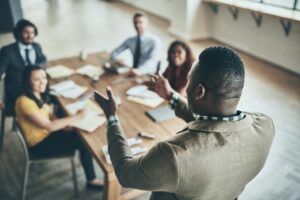
Empowering Your People Isn’t Enough | DConsulted
Learn why empowering employees alone doesn’t guarantee success. Discover how strategic alignment and leadership support drive real results.
In today’s fast-paced software engineering landscape, moving quickly from design models to functional code is more important than ever. Especially in safety-critical systems like automotive, aerospace, and medical devices, precision, rigor, and scalability are nonnegotiable. Unified Modeling Language (UML) diagrams have long been a cornerstone for ensuring traceability and consistency during the software life cycle.
Generative AI tools like ChatGPT are now revolutionizing this process. By interpreting UML diagrams and generating clean, structured code, these tools enable:
This blog explores how ChatGPT can streamline UML-to-code workflows, complete with a real-world example of generating modular C++ code from a UML diagram.
Listed below are the reasons why generative AI is useful for model-to-code transformation:
Generative AI tools like ChatGPT simplify the transition from models to code by automating mundane tasks. This allows developers to focus on problem-solving and optimization rather than writing repetitive code.
By translating UML diagrams into code systematically, AI minimizes the risk of errors caused by misinterpretation or oversight, ensuring alignment with architectural intent.
ChatGPT acts as a real-time assistant, capable of generating code snippets, debugging errors, and providing context-aware suggestions for improvements.
Whether your project uses C++, Python, or Java, ChatGPT adapts seamlessly, generating code in multiple programming languages.
While basic UML diagrams provide functionality descriptions, ChatGPT and other LLMs are getting better and better and can also work with sequence or activity diagrams for more complex workflows, offering deeper insights into the system’s behavior.
To understand better, discussed below is a simple example of UML-to-code:
Imagine a system where:
The system adheres to modular design principles using the Factory Pattern, ensuring scalability, traceability, and compliance with safety standards.
AI-generated code example
Below is an example of how ChatGPT translates UML models into structured C++ code.
#include
#include
#include
// Abstract base class: Request
class Request {
public:
virtual void execute() = 0; // Pure virtual function
virtual ~Request() = default;
};
// Concrete class: ResetRequest
class ResetRequest : public Request {
public:
void execute() override {
std::cout << "Executing Reset Request" << std::endl;
}
};
// Concrete class: ClearDTCRequest
class ClearDTCRequest : public Request {
public:
void execute() override {
std::cout << "Executing Clear DTC Request" << std::endl;
}
};
// Factory Class: RequestFactory
class RequestFactory {
public:
static std::unique_ptr create(const std::string& data) {
if (data == "Reset") {
return std::make_unique();
} else if (data == "ClearDTC") {
return std::make_unique();
} else {
return nullptr;
}
}
};
// Class: Service
class Service {
public:
bool validateData(const std::string& data) {
return !data.empty(); // Simple validation logic
}
void OnNewMessage(const std::string& data) {
if (validateData(data)) {
auto request = RequestFactory::create(data);
if (request) {
request->execute();
} else {
std::cout << "Invalid Request Type" << std::endl;
}
}
}
};
// Class: Connection
class Connection {
public:
void setOnMessageHandler(Service& service) {
// Simulate receiving messages
service.OnNewMessage("Reset");
service.OnNewMessage("ClearDTC");
service.OnNewMessage("InvalidRequest");
}
};
int main() {
Connection connection;
Service service;
connection.setOnMessageHandler(service);
return 0;
}
Listed below are the key advantages of AI-generated code:
By leveraging these advantages, ChatGPT significantly boosts efficiency and reliability, positioning itself as a valuable tool in modern software development workflows.
While ChatGPT accelerates the development process, safety-critical software demands thorough validation and verification:
Generative AI tools like ChatGPT are transforming software engineering, enabling faster, more accurate transitions from UML models to functional code. While the initial outputs may require refinement, these tools provide a structured foundation for creating safety-critical systems.
And this is just the beginning. The potential for optimizing AI-generated code is immense—future advancements will focus on leveraging modern C++ features, enhancing runtime performance, and reducing compilation overhead. Achieving high-performance, production-ready code through automated iteration, real-time feedback, and guided optimization will be a key part of this evolution.
Are you ready to revolutionize your development workflow? Try ChatGPT today to transform UML diagrams into functional code. Share your experiences and feedback with us – we’d love to hear about your real-world use cases!
For more insights into model-driven development and generative AI, check out our related resources.
Recent Articles
Learn why empowering employees alone doesn’t guarantee success. Discover how strategic alignment and leadership support drive real results.
AI-powered requirement review tools simplify engineering verification, ensuring accuracy, efficiency, and compliance with INCOSE guidelines. Learn more about it here.
Managing and optimizing thread overhead is important for safety-critical and embedded systems. Learn more about the C++ multithread common myths here.
Managing and optimizing thread overhead is important for safety-critical and embedded systems. Learn more about the C++ multithread common myths here.
Discover the critical role of effective interface management in complex systems. Learn how centralized tools, AI-powered solutions, and well-defined processes can prevent errors, enhance collaboration, and ensure safety in industries like automotive and aerospace.