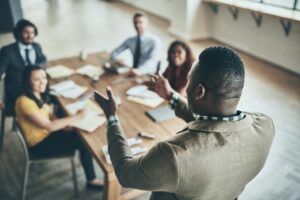
Empowering Your People Isn’t Enough | DConsulted
Learn why empowering employees alone doesn’t guarantee success. Discover how strategic alignment and leadership support drive real results.
Are you grappling with the complex coding guidelines essential for safety-critical software?
One rule that often leads to considerable confusion and frustration is the cautious use of reinterpret_cast. Astonishingly, some sources even recommend substituting reinterpret_cast with static_cast<void*> as a solution. Let’s delve into why this approach is not only insufficient but could also lead to perilous outcomes in your software development endeavors. In this article, we will explore two robust solutions to this challenge: one utilizing polymorphism for dynamic type safety and enhanced code clarity, and another leveraging templates for compile-time safety and performance optimization in embedded systems.
Using reinterpret_cast is a free for all use to convert any type to any type! A real danger in safety critical software! Understanding these risks is crucial for developing robust and reliable software:
Note on reinterpret_cast Usage: While this article cautions against the use of reinterpret_cast in safety-critical systems, it’s important to recognize that there are specific, rare scenarios where its use is justified. These include low-level system programming, interfacing with hardware, or dealing with legacy code where direct control over memory representation is essential. In such cases, reinterpret_cast should be used with extreme caution, accompanied by thorough documentation, rigorous testing, and strict safety checks to mitigate risks.
In addressing the challenges posed by reinterpret_cast and void*, it’s not just about enhancing safety; it’s also about adopting practices that lead to more efficient and easier-to-maintain code. Here are key alternatives that achieve these goals:
Consider handling different camera types like standard, infrared, and wide-angle in an ADAS system. A common approach might use reinterpret_cast for type switching, which is risky in safety-critical systems.
A common but unsafe approach might use reinterpret_cast to switch between camera data types:
#include
class StandardCamera {
public:
void captureStandard() { std::cout << "Capturing with Standard Camera" << std::endl; }
};
class InfraredCamera {
public:
void captureInfrared() { std::cout << "Capturing with Infrared Camera" << std::endl; }
};
class WideAngleCamera {
public:
void captureWideAngle() { std::cout << "Capturing with Wide Angle Camera" << std::endl; }
};
enum CameraType {
Standard,
Infrared,
WideAngle
};
void processCamera(void* rawCamera, CameraType cameraType) {
if (cameraType == Standard) {
auto* camera = reinterpret_cast(rawCamera);
camera->captureStandard();
} else if (cameraType == Infrared) {
auto* camera = reinterpret_cast(rawCamera);
camera->captureInfrared();
} else if (cameraType == WideAngle) {
auto* camera = reinterpret_cast(rawCamera);
camera->captureWideAngle();
}
}
int main() {
StandardCamera stdCam;
InfraredCamera irCam;
WideAngleCamera waCam;
processCamera(&stdCam, Standard);
processCamera(&irCam, Infrared);
processCamera(&waCam, WideAngle);
}
In the provided code example, processCamera function uses reinterpret_cast to cast a void* to specific camera types based on an integer identifier. This approach is fraught with risks, particularly in safety-critical systems:
We can refactor this using polymorphism, eliminating reinterpret_cast:
#include
class Camera {
public:
virtual ~Camera() = default;
virtual void capture() = 0;
};
class StandardCamera : public Camera {
public:
void capture() override {
captureStandard();
}
void captureStandard() { std::cout << "Capturing with Standard Camera" << std::endl; }
};
class InfraredCamera : public Camera {
public:
void capture() override {
captureInfrared();
}
void captureInfrared() { std::cout << "Capturing with Infrared Camera" << std::endl; }
};
class WideAngleCamera : public Camera {
public:
void capture() override {
captureWideAngle();
}
void captureWideAngle() { std::cout << "Capturing with Wide Angle Camera" << std::endl; }
};
void processCamera(std::unique_ptr camera) {
camera->capture();
}
int main() {
std::unique_ptr stdCam = std::make_unique();
std::unique_ptr irCam = std::make_unique();
std::unique_ptr waCam = std::make_unique();
processCamera(std::move(stdCam));
processCamera(std::move(irCam));
processCamera(std::move(waCam));
}
The refactored code demonstrates a safer and more robust approach to handling different camera types in a safety-critical system:
For performance-critical embedded systems, templates offer a solution:
#include
class StandardCamera {
public:
void capture() {
std::cout << "Capturing with Standard Camera" << std::endl;
}
};
class InfraredCamera {
public:
void capture() {
std::cout << "Capturing with Infrared Camera" << std::endl;
}
};
class WideAngleCamera {
public:
void capture() {
std::cout << "Capturing with Wide Angle Camera" << std::endl;
}
};
template
void processCamera(CameraType& camera) {
camera.capture();
}
int main() {
StandardCamera stdCam;
InfraredCamera irCam;
WideAngleCamera waCam;
processCamera(stdCam);
processCamera(irCam);
processCamera(waCam);
}
The updated example showcases an optimized approach using templates, which is particularly beneficial for performance-sensitive systems like automotive ADAS (Advanced Driver-Assistance Systems):
When implementing templates in Advanced Driver-Assistance Systems (ADAS) for camera processing, there are several key considerations and trade-offs to keep in mind:
Moving away from reinterpret_cast in safety-critical C++ systems is not just about adhering to best practices; it’s a fundamental shift towards ensuring robustness and reliability. By embracing class hierarchies and templates, we significantly reduce the risks associated with unsafe type conversions, paving the way for more secure and stable software architectures.
In Conclusion:
The journey from the risky terrains of reinterpret_cast to the safer realms of polymorphism and templates is a testament to the evolving nature of C++ programming, especially in safety-critical environments. While reinterpret_cast might offer a quick fix, its potential for introducing type safety issues and maintenance challenges is a significant concern.
Our exploration underscores two powerful alternatives:
Adopting these strategies marks a commitment to developing software that is not only functionally robust but also adheres to the highest standards of safety and reliability. For developers and teams dedicated to excellence in safety-critical software, this shift is not just a recommendation – it’s an imperative.
Will you settle for marginal average software or the best of the best? Most settle for average.
Other Articles
Learn why empowering employees alone doesn’t guarantee success. Discover how strategic alignment and leadership support drive real results.
Generative AI tools like ChatGPT are revolutionizing software engineering by converting UML diagrams into accurate, structured code. Learn more about it here.
AI-powered requirement review tools simplify engineering verification, ensuring accuracy, efficiency, and compliance with INCOSE guidelines. Learn more about it here.
Managing and optimizing thread overhead is important for safety-critical and embedded systems. Learn more about the C++ multithread common myths here.
Managing and optimizing thread overhead is important for safety-critical and embedded systems. Learn more about the C++ multithread common myths here.
Discover the critical role of effective interface management in complex systems. Learn how centralized tools, AI-powered solutions, and well-defined processes can prevent errors, enhance collaboration, and ensure safety in industries like automotive and aerospace.
The system requirements analysis process under ASPICE and ISO26262 frameworks shapes automotive system architecture design for enhanced safety, reliability, and compliance. Learn more about it here.
Compliance with ASPICE and ISO26262 standards provides a significant impact on automotive software development. Learn more about it here.
AI technologies like ChatGPT-4 are revolutionizing requirements engineering by improving accuracy, consistency, and efficiency. Learn about the role of AI in refining, validating, and managing project requirements here.
Model-Based Systems Engineering (MBSE) offers a robust framework to streamline the entire engineering process, from design to validation. Learn more about it here.
Data-Oriented Design (DOD) can revolutionize automotive software development by enhancing performance, reducing costs, and minimizing hardware requirements. Learn more about it here.
Explore the evolution of functional safety, its growing importance in industries like automotive, and the critical role of Fault Tolerant Time Interval (FTTI) in ensuring system reliability. Learn how FTTI, along with Malfunctioning Behavior Manifestation Time (MBMT) and Hazard Manifestation Time (HMT), contributes to robust safety designs, preventing hazards in safety-related systems such as ADAS and autonomous vehicles. Discover the impact of ISO 26262 standards on the development of effective fault detection and reaction mechanisms in automotive safety.
While ISO 26262 primarily addresses functional safety, SOTIF extends the scope to focus on potential hazards arising from system behaviour, even when the system functions as intended. Learn more about it here.
In a rapidly evolving technological landscape, the demand for systems that can not only withstand errors but also adapt to them is paramount. This article delves into the world of Fault-Tolerant (FT) systems, emphasizing their significance in maintaining the functionality and safety of critical operations across various sectors. It explores the latest advancements in FT technology, underscoring the importance of resilience and adaptability in ensuring uninterrupted service and safeguarding against potential failures.
In a rapidly evolving technological landscape, the demand for systems that can not only withstand errors but also adapt to them is paramount. This article delves into the world of Fault-Tolerant (FT) systems, emphasizing their significance in maintaining the functionality and safety of critical operations across various sectors. It explores the latest advancements in FT technology, underscoring the importance of resilience and adaptability in ensuring uninterrupted service and safeguarding against potential failures.
Discover the transformative impact of Software-Defined Networking (SDN) and Multicast Addressing on automotive embedded systems. Explore how these technologies enhance communication efficiency, safety, and performance in the automotive industry, leading to cost-effective, scalable, and eco-friendly solutions. Dive into the technical advantages and practical applications for modern vehicles and infrastructure.
Discover how ChatGPT revolutionizes engineering with AI, accelerating learning, enhancing safety, and boosting productivity.
Defect Escape Reduction Rate and feedback loop elevate testing. Learn more about them here.
ASPICE and ISO26262 frameworks improve system development in the automotive industry, ensuring safety, compliance, and high-quality standards.
Conducting software FMEA, FTA, and compliance with ISO 26262 helps developers create software that meets stringent safety requirements. Learn more about it here.
Explore the challenges of dynamic memory allocation in critical software, learn how to mitigate failures, and ensure the reliability of safety-critical systems. Real-life failures and practical solutions are discussed.
Strong types are a key concept in C++ programming for functional safety. Learn how strong types can reduce errors in critical systems with C++.
Unit testing is an essential part of the software development process. Learn more about it here.
Learn about categorizing requirements, including functional, non-functional, performance, interface etc for effective system management and development.